To get the screen dpi using wxPython, you can use the wx.ScreenDC()
method to create a device context for the screen. Then, you can use the GetPPI()
method on the device context object to retrieve the screen's resolution in dots per inch (dpi). This will give you the horizontal and vertical dpi values of the screen. You can then use these values to calculate the overall screen dpi if needed.
Best Python Books to Read in December 2024
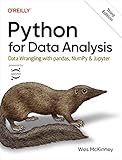
Rating is 4.9 out of 5
Python for Data Analysis: Data Wrangling with pandas, NumPy, and Jupyter
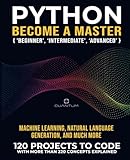
Rating is 4.7 out of 5
Python Practice Makes a Master: 120 ‘Real World’ Python Exercises with more than 220 Concepts Explained (Mastering Python Programming from Scratch)
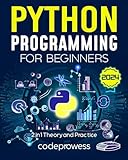
Rating is 4.6 out of 5
Python Programming for Beginners: The Complete Python Coding Crash Course - Boost Your Growth with an Innovative Ultra-Fast Learning Framework and Exclusive Hands-On Interactive Exercises & Projects
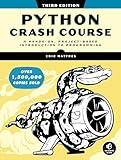
Rating is 4.4 out of 5
Python Crash Course, 3rd Edition: A Hands-On, Project-Based Introduction to Programming
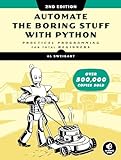
Rating is 4.3 out of 5
Automate the Boring Stuff with Python, 2nd Edition: Practical Programming for Total Beginners
What is the best method for getting the screen dpi in wxpython?
One of the best methods for getting the screen DPI in wxPython is by using the wx.Display.GetGeometry() method. This method returns a list of display dimensions, including the screen DPI. Here is an example code snippet that demonstrates how to get the screen DPI using this method:
1 2 3 4 5 6 7 8 |
import wx app = wx.App() display = wx.Display() geometry = display.GetGeometry() dpi = geometry.GetPPI() print("Screen DPI:", dpi) |
This code snippet creates a wx.Display object and uses the GetGeometry() method to get the display dimensions, including the DPI. The DPI is then accessed using the GetPPI() method, which returns a tuple containing the width and height DPI values. Finally, the DPI values are printed to the console.
How to calculate the screen dpi in wxpython?
To calculate the screen dpi in wxPython, you can use the following code:
1 2 3 4 5 6 |
import wx app = wx.App(False) dc = wx.ScreenDC() dpi = dc.GetPPI() print(f"Screen DPI: {dpi}") |
This code creates a wx.App object, initializes a wx.ScreenDC object to get the screen device context, and then calls the GetPPI() method to get the screen DPI. Finally, it prints out the screen DPI.
What is the default screen dpi value in wxpython?
The default screen dpi value in wxPython is typically 96 dpi (dots per inch).