To ignore a character followed by random numbers using regex, you can use the following pattern:
1
|
\w\d+
|
This pattern will match an alphanumeric character followed by one or more digits. You can use this pattern in your regular expression to ignore such characters with random numbers.
Best Software Engineering Books of June 2025
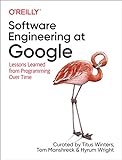
Rating is 5 out of 5
Software Engineering at Google: Lessons Learned from Programming Over Time
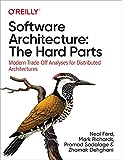
Rating is 4.9 out of 5
Software Architecture: The Hard Parts: Modern Trade-Off Analyses for Distributed Architectures
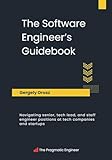
Rating is 4.8 out of 5
The Software Engineer's Guidebook: Navigating senior, tech lead, and staff engineer positions at tech companies and startups
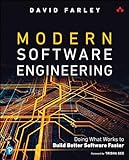
Rating is 4.7 out of 5
Modern Software Engineering: Doing What Works to Build Better Software Faster
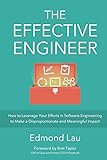
Rating is 4.5 out of 5
The Effective Engineer: How to Leverage Your Efforts In Software Engineering to Make a Disproportionate and Meaningful Impact
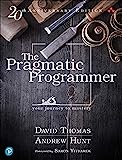
Rating is 4.2 out of 5
The Pragmatic Programmer: Your Journey To Mastery, 20th Anniversary Edition (2nd Edition)
What is the best approach for handling cases where characters may or may not have random numbers in regex?
One approach for handling cases where characters may or may not have random numbers in a regex is to use the "\d?" quantifier to match zero or one occurrence of a digit. This allows for flexibility in matching patterns that may or may not include random numbers.
For example, if you are looking to match a pattern like "abc123" or "abc" where the numbers are optional, you can use the regex pattern "abc\d?".
Another approach is to use character classes to specify a range of acceptable characters. For example, if you want to match any alphanumeric character followed by one or more digits, you can use the pattern "[a-zA-Z]+\d+".
Additionally, using grouping and alternation can be useful for handling cases where characters may or may not have random numbers in regex. For example, the pattern "(abc)?\d{3}" will match either "abc123" or "123" where "abc" is optional.
Overall, the best approach for handling cases where characters may or may not have random numbers in regex will depend on the specific requirements of the pattern you are trying to match. Experimenting with different techniques and patterns can help you find the most effective solution for your particular regex scenario.
What is the role of flags in modifying regex behavior when ignoring random numbers?
Flags in regular expressions are used to modify the behavior of the regex pattern matching. When ignoring random numbers, the "g" flag can be used in JavaScript regex to perform a global search which searches for all occurrences in a string instead of stopping after the first match.
For example, a regex pattern like /[a-z]+/g
with the "g" flag would match all sequences of lowercase letters in a string, ignoring any random numbers present.
How to match characters but ignore those with random numbers using regex?
You can use the following regex pattern to match characters but ignore those with random numbers:
1
|
\b[A-Za-z]+\b
|
This pattern will only match sequences of alphabetical characters (both uppercase and lowercase) surrounded by word boundaries. It will ignore any sequences that contain numbers or other special characters.