In Golang, you can get the current year using the time
package. Here's an example of how to achieve this:
1 2 3 4 5 6 7 8 9 10 11 |
package main import ( "fmt" "time" ) func main() { currentYear := time.Now().Year() fmt.Println("Current Year:", currentYear) } |
In this code, the time.Now()
function returns the current time, and Year()
extracts the year from it. Finally, fmt.Println()
is used to print the current year.
Best Golang Books to Read in 2024
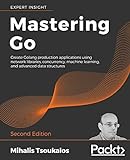
Rating is 5 out of 5
Mastering Go: Create Golang production applications using network libraries, concurrency, machine learning, and advanced data structures, 2nd Edition
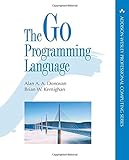
Rating is 4.9 out of 5
Go Programming Language, The (Addison-Wesley Professional Computing Series)
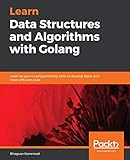
Rating is 4.8 out of 5
Learn Data Structures and Algorithms with Golang: Level up your Go programming skills to develop faster and more efficient code
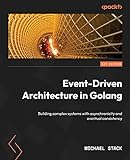
Rating is 4.7 out of 5
Event-Driven Architecture in Golang: Building complex systems with asynchronicity and eventual consistency
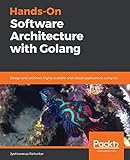
Rating is 4.6 out of 5
Hands-On Software Architecture with Golang: Design and architect highly scalable and robust applications using Go
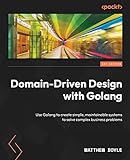
Rating is 4.5 out of 5
Domain-Driven Design with Golang: Use Golang to create simple, maintainable systems to solve complex business problems
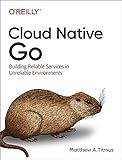
Rating is 4.3 out of 5
Cloud Native Go: Building Reliable Services in Unreliable Environments
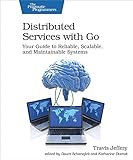
Rating is 4.2 out of 5
Distributed Services with Go: Your Guide to Reliable, Scalable, and Maintainable Systems
What is the method for getting the year in Golang's time package?
To get the current year using the time package in Go, you can use the Now()
function from the time package along with Year()
method. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 |
package main import ( "fmt" "time" ) func main() { currentYear := time.Now().Year() fmt.Println(currentYear) } |
The time.Now()
function returns the current time and date as a Time
struct, and then we use the Year()
method to extract the year from that struct. Finally, we can print the current year using fmt.Println()
.
What is the correct way to access the year component of the current time in Golang?
In Go, we can access the year component of the current time using the time.Now()
function from the time
package. Here's an example of how to do it correctly:
1 2 3 4 5 6 7 8 9 10 11 12 |
package main import ( "fmt" "time" ) func main() { currentTime := time.Now() year := currentTime.Year() fmt.Println(year) } |
By calling time.Now()
, we get the current time as a time.Time
object, and then we can use the Year()
method on that object to extract the year component.
How do I access the year component of the current time in Golang using the time package?
You can access the year component of the current time in Golang using the time package by calling the Now()
function to get the current time and then using the Year()
method to extract the year component.
Here's an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
package main import ( "fmt" "time" ) func main() { currentTime := time.Now() year := currentTime.Year() fmt.Println("Current Year:", year) } |
In this example, time.Now()
returns the current time, and then we call the Year()
method on the resulting Time
value to extract the year component. Finally, the value of the year component is printed using fmt.Println()
.
Best Golang Hosting Providers of July 2024
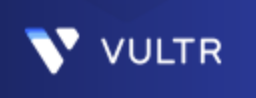
Rating is 5 out of 5
Vultr
- Ultra-fast Intel Core Processors
- Great Uptime and Support
- High Performance and Cheap Cloud Dedicated Servers

Rating is 4.9 out of 5
Digital Ocean
- Professional hosting starting at $5 per month
- Remarkable Performance
What is the syntax for extracting the year from the current time in Golang?
To extract the year from the current time in Golang, you can use the time.Now()
function to get the current time, and then use the Year()
method to extract the year. Here is an example of the syntax:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
package main import ( "fmt" "time" ) func main() { currentTime := time.Now() year := currentTime.Year() fmt.Println("Current Year:", year) } |
In this example, the time.Now()
function is used to get the current time, and currentTime.Year()
is used to extract the year from the current time. The Year()
method returns the year as an integer. Finally, the result is printed using fmt.Println()
.
How to retrieve the year from the current timestamp using Golang's time package?
To retrieve the year from the current timestamp using Golang's time package, you can use the Now() function from the time package to get the current time and then use the Year() method to get the year. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 12 |
package main import ( "fmt" "time" ) func main() { currentTime := time.Now() year := currentTime.Year() fmt.Println(year) } |
In this example, we first call the Now() function to get the current time. Then we use the Year() method on the currentTime variable to extract the year. Finally, we print the year using fmt.Println().
When you run this code, it will output the current year from the current timestamp.