To get the length of a string in Swift, you can use the count
method on the string variable. This method returns the number of characters in the string, including any white spaces or special characters. Simply call yourString.count
to get the length of the string.
Best Swift Books to Read of April 2025
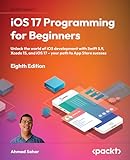
Rating is 4.8 out of 5
iOS 17 Programming for Beginners - Eighth Edition: Unlock the world of iOS Development with Swift 5.9, Xcode 15, and iOS 17 - Your Path to App Store Success
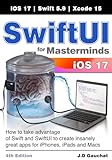
Rating is 4.7 out of 5
SwiftUI for Masterminds 4th Edition: How to take advantage of Swift and SwiftUI to create insanely great apps for iPhones, iPads, and Macs
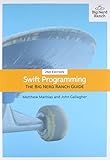
Rating is 4.5 out of 5
Swift Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
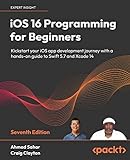
Rating is 4.4 out of 5
iOS 16 Programming for Beginners: Kickstart your iOS app development journey with a hands-on guide to Swift 5.7 and Xcode 14, 7th Edition
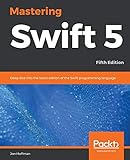
Rating is 4.3 out of 5
Mastering Swift 5: Deep dive into the latest edition of the Swift programming language, 5th Edition
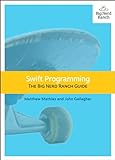
Rating is 4.2 out of 5
Swift Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
How to declare a string variable in Swift?
To declare a string variable in Swift, you can use the following syntax:
1
|
var myString: String = "Hello, World!"
|
In this example, the variable myString
is declared as a String type and assigned the value "Hello, World!". You can also declare a string variable without explicitly specifying the type, as Swift automatically infers the type based on the assigned value:
1
|
var myString = "Hello, World!"
|
How to convert a string to a data object in Swift?
You can convert a string to a data object in Swift by using the data(using:)
method available on the String class. Here's an example of how you can do it:
1 2 3 4 5 6 7 |
let string = "Hello, World!" if let data = string.data(using: .utf8) { // Use the data object print(data) } else { print("Failed to convert string to data") } |
In this example, the data(using:)
method is called on the string "Hello, World!"
with the parameter .utf8
specifying the encoding to be used. If the conversion is successful, the data object will be stored in the data
variable. If the conversion fails, the code will print a message indicating the failure.
What is a string slicing in Swift?
String slicing in Swift refers to the process of extracting a substring from a given string based on a specified range of indices. This operation allows you to work with a portion of a string rather than the entire string itself. You can use the slicing syntax to specify the start and end indices of the substring you want to extract.