In order to replace the ticks in matplotlib, you can use the set_xticks() and set_yticks() functions to customize the ticks on the x and y axes, respectively. You can pass a list of values to these functions to set the specific ticks you want to display. Additionally, you can use set_xticklabels() and set_yticklabels() to specify the labels for the ticks. This allows you to replace the default ticks with custom values or labels of your choosing.
Best Python Books to Read in November 2024
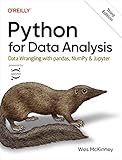
Rating is 4.9 out of 5
Python for Data Analysis: Data Wrangling with pandas, NumPy, and Jupyter
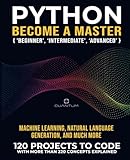
Rating is 4.7 out of 5
Python Practice Makes a Master: 120 ‘Real World’ Python Exercises with more than 220 Concepts Explained (Mastering Python Programming from Scratch)
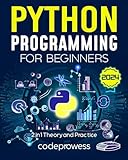
Rating is 4.6 out of 5
Python Programming for Beginners: The Complete Python Coding Crash Course - Boost Your Growth with an Innovative Ultra-Fast Learning Framework and Exclusive Hands-On Interactive Exercises & Projects
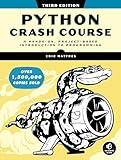
Rating is 4.4 out of 5
Python Crash Course, 3rd Edition: A Hands-On, Project-Based Introduction to Programming
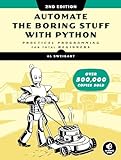
Rating is 4.3 out of 5
Automate the Boring Stuff with Python, 2nd Edition: Practical Programming for Total Beginners
What is the default tick direction in matplotlib?
The default tick direction in matplotlib is usually inward.
How to remove ticks in matplotlib?
To remove ticks in matplotlib, you can use the following code:
1 2 3 4 5 6 |
import matplotlib.pyplot as plt plt.plot([1, 2, 3, 4]) plt.xticks([]) # This line will remove the x-axis ticks plt.yticks([]) # This line will remove the y-axis ticks plt.show() |
This code will create a simple plot with no ticks on the x and y axes. You can also customize the tick positions, labels, and other properties using various functions provided by matplotlib.
How to hide ticks on a specific axis in matplotlib?
To hide ticks on a specific axis in matplotlib, you can use the set_ticks
method of the Axis object for that specific axis. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import matplotlib.pyplot as plt # Create a figure and axis fig, ax = plt.subplots() # Plot some data x = [1, 2, 3, 4, 5] y = [10, 20, 30, 40, 50] ax.plot(x, y) # Hide ticks on the x-axis ax.xaxis.set_ticks([]) plt.show() |
In this example, we created a figure and axis, plotted some data, and then used the set_ticks
method on the x-axis to hide the ticks. You can do the same for the y-axis by using ax.yaxis.set_ticks([])
.